Working with XMP Metadata¶
Retrieving XMP data¶
In raster images, XMP data exist at the frame level. Here is how to extract the XMP data from the first frame:
C_TEXT($rasterDocRef) // The raster document reference // ... C_TEXT($xmpDataRef) $xmpDataRef:=RasterFrame_CopyXMPData ($rasterDocRef;1) If ($xmpDataRef#"") // ... // work with $xmpDataRef // ... // Release the XMP data when no longer needed ImgObj_Release ($xmpDataRef) End if
In PDF documents, XMP data are at the document level:
C_TEXT($pdfDocRef) // The PDF document reference // ... C_TEXT($xmpDataRef) $xmpDataRef:=PDFDoc_CopyXMPData ($pdfDocRef) If ($xmpDataRef#"") // ... // work with $xmpDataRef // ... // Release the XMP data when no longer needed ImgObj_Release ($xmpDataRef) End if
Read properties¶
Reading property values from XMP data is done via dedicated methods:
C_TEXT($xmpDataRef) // The XMP data reference // ... // exif:ImageUniqueID is a 'simple' property C_TEXT(gImageID) gImageID:=XMPData_GetSimplePropertyValue ($xmpDataRef;"exif:ImageUniqueID") // dc:publisher is a 'bag' property ARRAY TEXT($publisher;0) XMPData_GetArrayPropertyItems ($xmpDataRef;"dc:publisher";->$publisher) // exif:Flash/exif:Fired is a nested 'simple' property with 'boolean' value C_BOOLEAN($flashFired) $flashFired:=(XMPData_GetSimplePropertyValue ($xmpDataRef;"exif:Flash/exif:Fired")="True")
Modify properties¶
Simple XMP properties are assigned data with the XMPData_SetSimplePropertyValue
method:
C_TEXT($xmpDataRef) // The XMP data reference C_TEXT($imageID) // The image ID C_TEXT($imageCreator) // ... XMPData_SetSimplePropertyValue ($xmpDataRef;"dc:identifier";$imageID) // dc:creator is an array property, set the first item. XMPData_SetSimplePropertyValue ($xmpDataRef;"dc:creator[1]";$imageCreator)
LangAlt
XMP properties are assigned data with the XMPData_SetLangAltPropValue
method:
C_TEXT($xmpDataRef) // The XMP data reference // ... XMPData_SetLangAltPropValue ($xmpDataRef;"dc:description";"en-US";"The image description") XMPData_SetLangAltPropValue ($xmpDataRef;"dc:description";"fr-FR";"La description de l'image")
In XMP data packets empty properties are removed. For example, the following calls are equivalent:
C_TEXT($xmpDataRef) // The XMP data reference // ... XMPData_SetSimplePropertyValue ($xmpDataRef;"dc:identifier";"") XMPData_RemoveProperty ($xmpDataRef;"dc:identifier")
Assigning XMP data¶
To assign XMP data to a raster frame do the following:
C_TEXT($rasterDocRef) // The raster document reference C_TEXT($xmpDataRef) // The XMP data reference // ... // Assign $xmpDataRef to the first frame RasterFrame_SetXMPData ($rasterDocRef;1;$xmpDataRef)
XMP data and RDF¶
XMP data are encoded internally as RDF.
It is possible to both serialize XMP data to RDF and create XMP data from RDF. This may be useful for saving XMP data in sidecar files alongside images or other kinds of documents that do not support XMP data natively.
To save XMP data to an RDF file:
C_TEXT($xmpDataRef) // The XMP data reference C_TEXT($rdfFilePath) // The file path of the RDF file // ... C_TEXT($rdf) $rdf:=XMPData_GetRDF ($xmpDataRef) TEXT TO DOCUMENT($rdfFilePath;$rdf)
To load XMP data from an RDF file:
C_TEXT($rdfFilePath) // The file path of the RDF file // ... C_TEXT($rdf) $rdf:=Document to text($rdfFilePath) If ($rdf#"") C_TEXT($xmpDataRef) $xmpDataRef:=XMPData_CreateWithRDF ($rdf) // ... // Work with $xmpDataRef // ... ImgObj_Release ($xmpDataRef) End if
Dumping the XMP data¶
Dumping may come handy while inspecting and understanding the structure of XMP data packets:
C_TEXT($xmpDataRef) // The XMP data reference C_TEXT($xmpDumpPath) // The path name of the dump file // ... C_TEXT($dump) $dump:=XMPData_Dump ($xmpDataRef) TEXT TO DOCUMENT($xmpDumpPath;$dump)
The generic XMP metadata editor¶
The Q2XMPLib component provides a generic metadata editor:
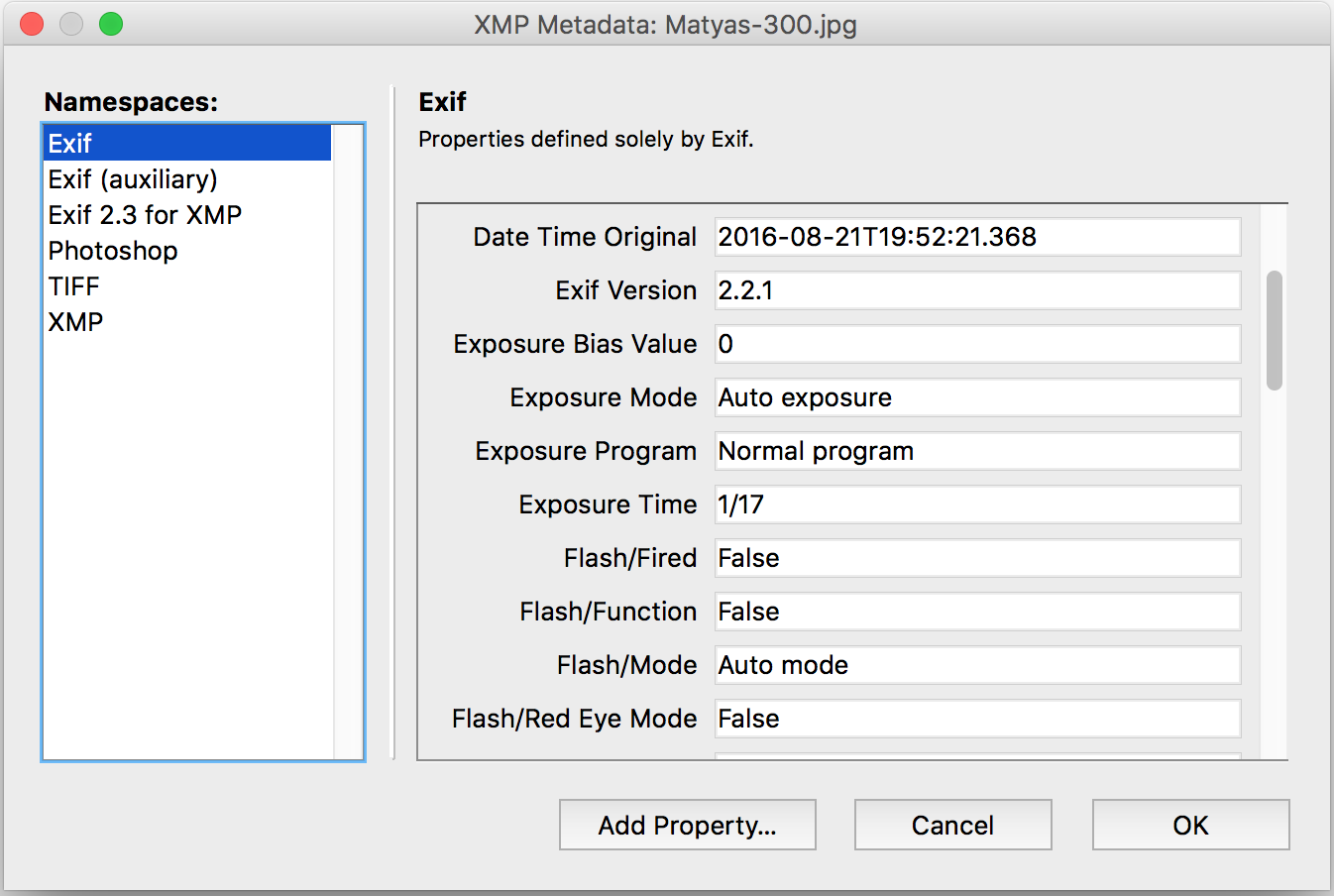
The editor is completely data-driven: namespace properties and their respective data types are specified in definition files in JSON format. The editor uses the property definitions to control property representation via appropriate 4D form objects, formatting, and editability according to the specification.
Here a new property is being added to the XMP data:
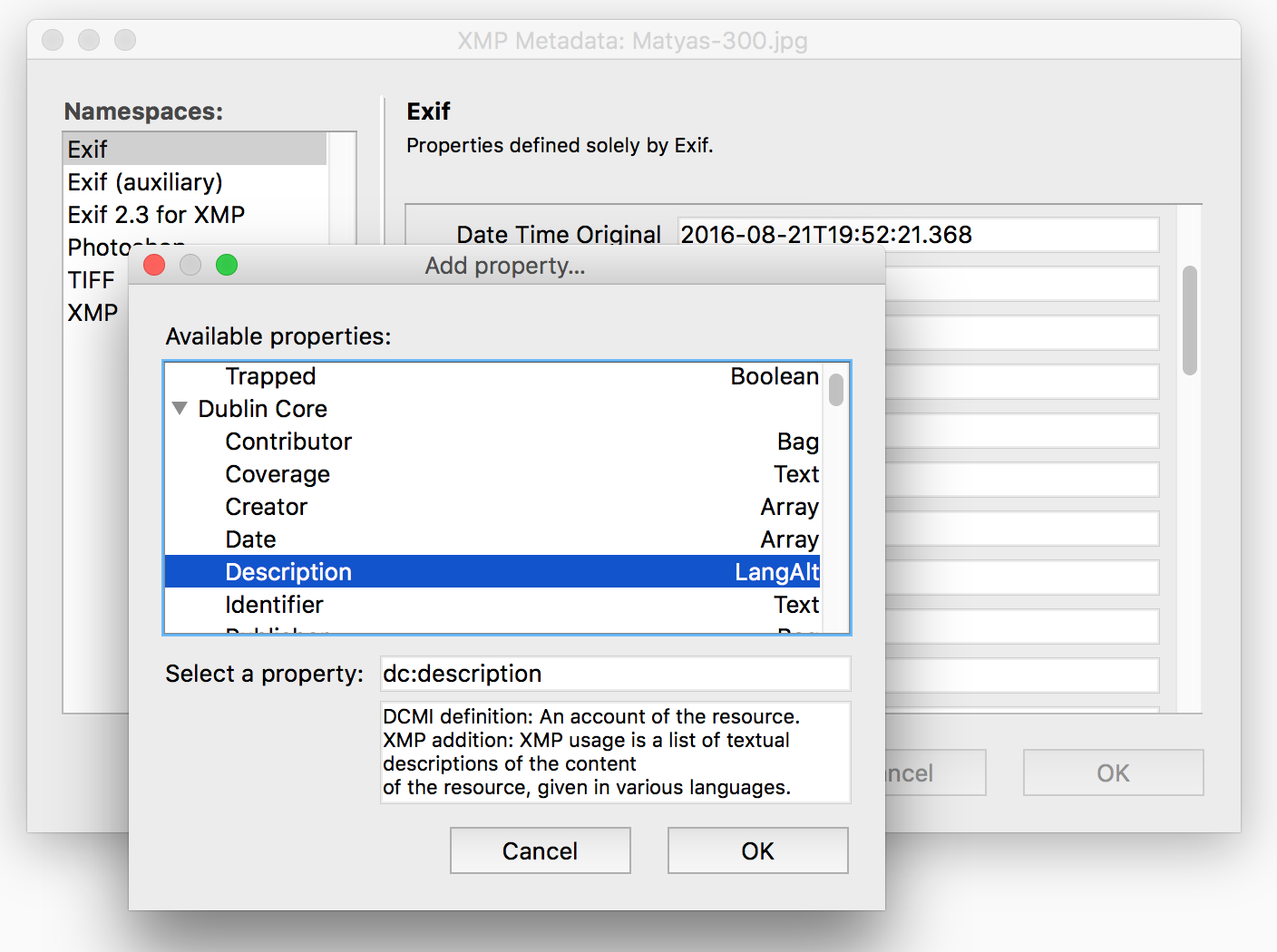
For properties of type LangAlt
a listbox with two columns (Language/Value) is used along with controls
for adding and removing languages:
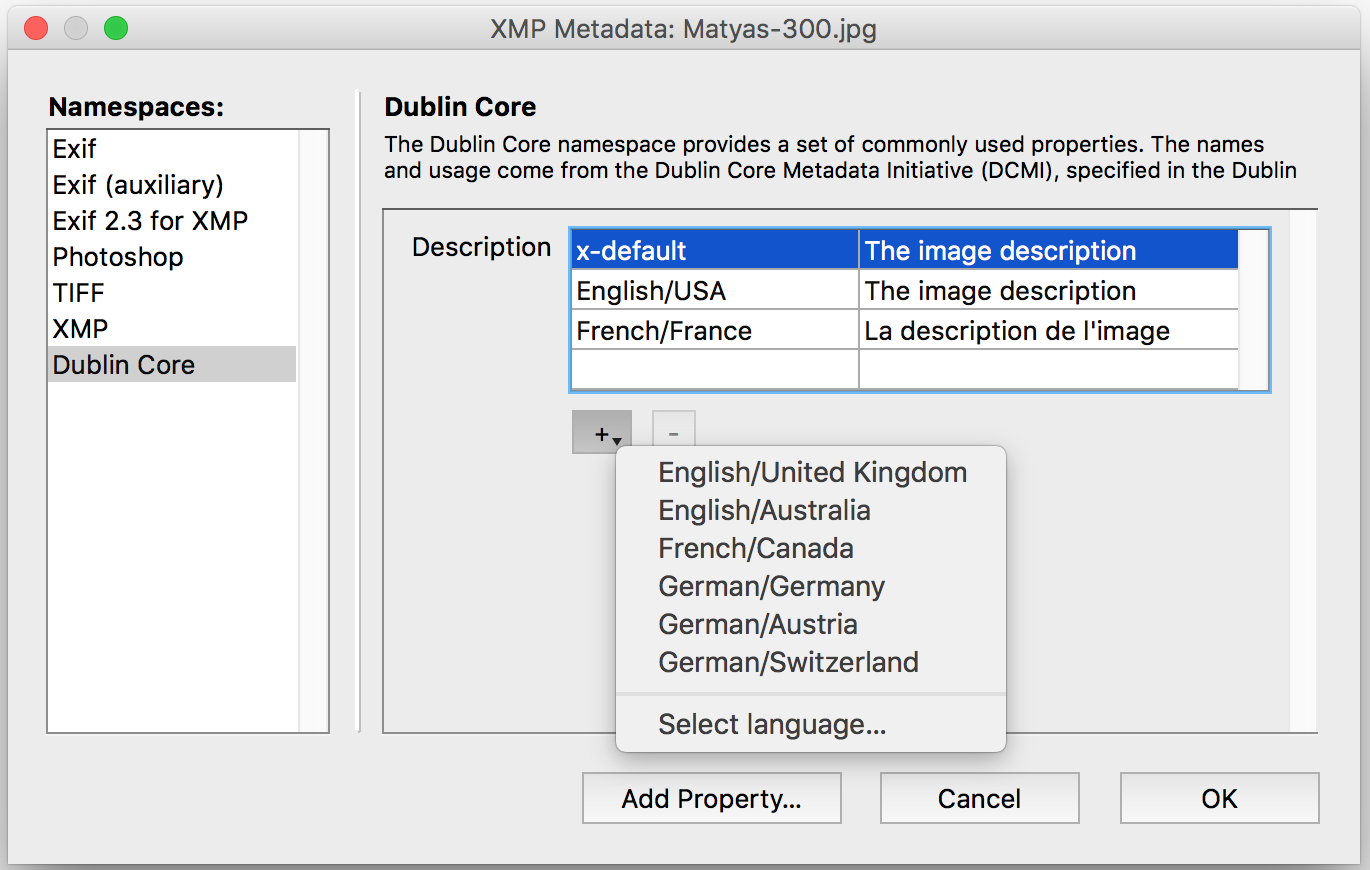
The above screeenshots show the XMP Editor running in end-user mode. The editor may also be invoked in developer mode, which provides several aids for understanding the structure of the XMP data:
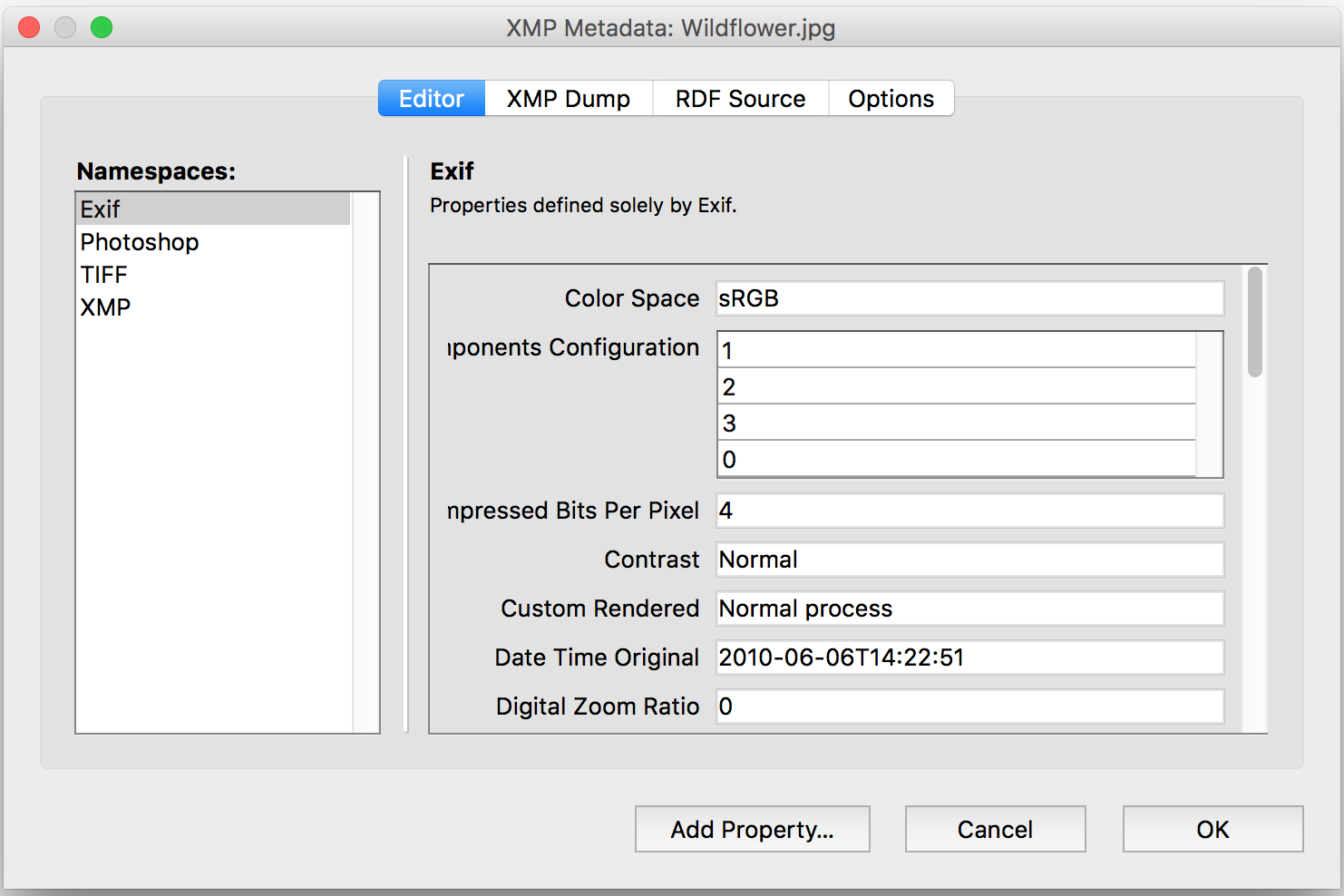
A textual dump of the XMP data:
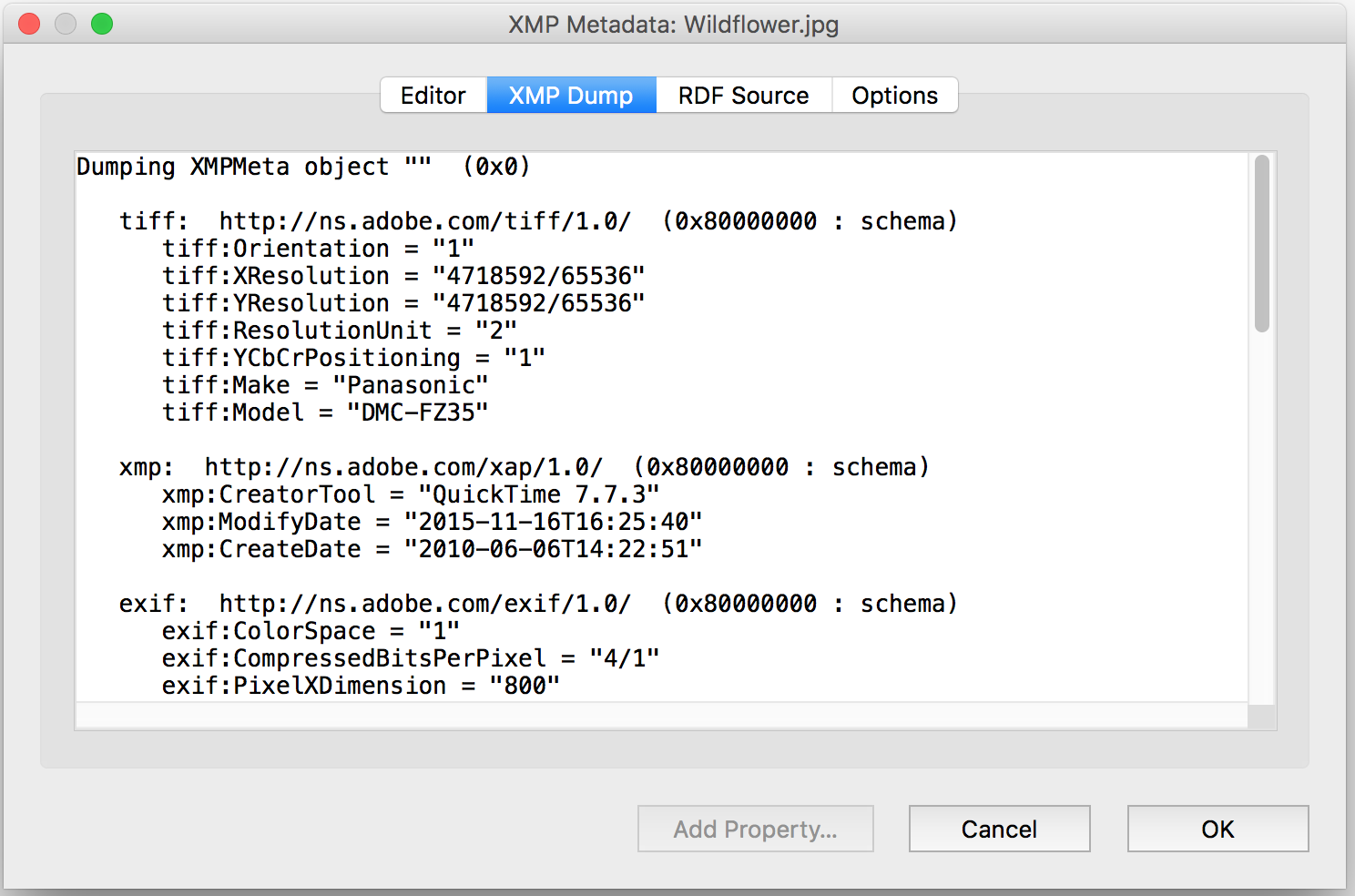
The RDF source of the XMP data:
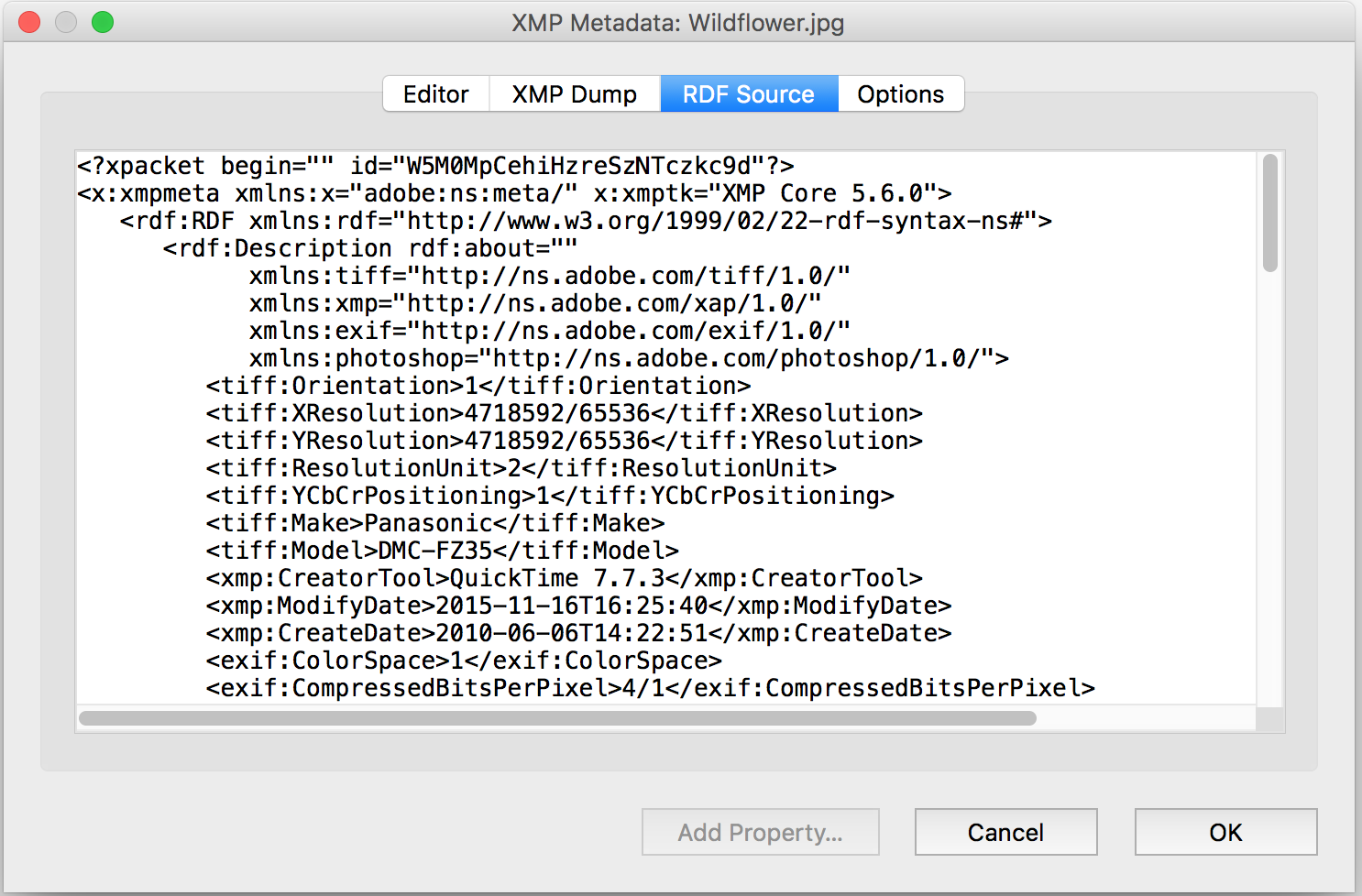
Options that control property editability:
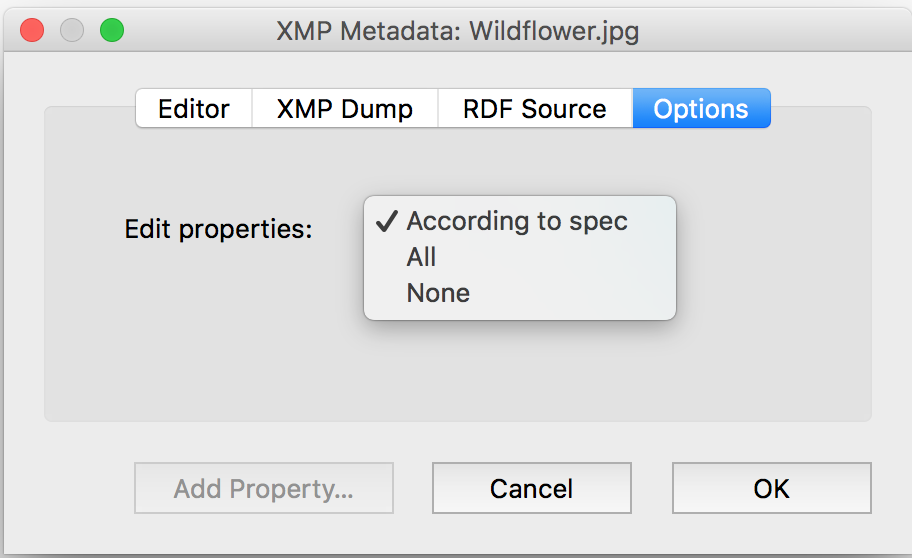
To invoke the XMP editor, call XMPDlog_EditData
.
Here the XMP data of the first frame of a raster image is presented to the user for editing. If the user validates the editor, the updated XMP data is assigned back to the raster image frame:
C_TEXT($rasterDocRef) // The raster document reference // ... C_TEXT($xmpDataRef) $xmpDataRef:=RasterFrame_CopyXMPData ($rasterDocRef;1) If ($xmpDataRef#"") C_LONGINT($success) $success:=XMPDlog_EditData ($xmpDataRef) If ($success=1) RasterFrame_SetXMPData ($docRef;$1;$xmpDataRef) End if // Release the XMP data when no longer needed ImgObj_Release ($xmpDataRef) End if
Running the XMP editor in developer mode is just a matter of passing the appropriate option
to XMPDlog_EditData
:
C_TEXT($xmpDataRef) // The XMP data reference // ... C_OBJECT($options) OB SET($options;"DevMode";True) C_LONGINT($success) $success:=XMPDlog_EditData ($xmpDataRef;$options) If ($success=1) // ... End if
Extending the XMP metadata editor¶
The XMP metadata editor can be extended by means of namespace and property definition JSON files. The format of these files will be finalized and documented in a subsequent beta release.